In this article, you will learn the most useful git commands that i have been using through my five years of experience as a software engineer.
While i know that there are some IDE that already propose an interface to manage those commands without typing it, i also know that knowing those command help me a lot to debug my git repositories (yes there can be some problems while dealing with a git repo) and to manage it because i don’t want the IDE to launch some commands behind the scene.
If you want to install git on your local machine, click here.
Configure your git
How to know which version of git you use.
git version
How to configure your git credentials (https access) on your local machine:
git config --global user.name "your name" git config --global user.email "your email address"
When you will try to clone a repo, git will ask for your password (github, gitlab, bitbucket or another one).
How to list all your git config :
git config -l
Create a git repository
How to create a git repository from an existing project.
- Create a remote project repository on your git account (gitlab, github)
- Copy that repositoy url (from the clone button): you can copy the ssh or the https url based on the authentification method that you configure on your local machine.
- Init a git project from your existing project: this command will init a git project with “master” as the default branch.
git init
- Set the remote url :
git remote add origin <my_url_repository> #It can be th https or the sh url
- Push your project on your remote git account : if the “master” branch doesn’t exist (in that case, the default branch will be “main” on your repository but you change it in your project settings), you will be asked to push the master branch as a new branch.
git push #use to push to an existing branch git push -u origin <my_New_branch> #Use to push a new branch. "-u" is the shortcut of --set-upstream
Git commands to manage a project
How to get a remote git repository on your local machine.
git clone <my_Url_Project> #It can be the https or the sh url
How to switch to another branch
git checkout <another_branch_name>
How to create a new branch on your local machine
git checkout -b <my_new_branch_name> # It Will create the new branch and switch on it
How to update your local branch from the remote branch
git pull
How to update your remote branch metadata
This command will only update the metadata of your remote branch locally. You can know if there has been some modification on that branch before pulling it. It will be available at origin/myBranchName.
git fetch
How to add new or modified files to a staging area.
git add <specified path to a file> #if you want to only add a file git add . #if you want to add all the new or modified files.
How to commit your modification to your local git repository.
This command is used after the “git add” command.
git commit -m "Description of what you want commit"
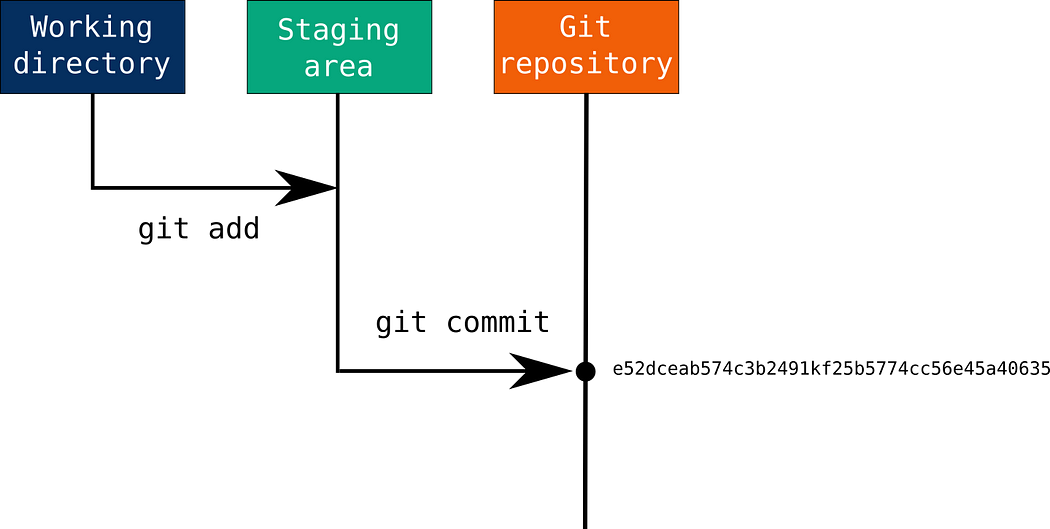
How to know the status of your repository.
It will help you to know what has been modified or create; What has been added or not; if you have an unpush commit etc.
git status #you can use this command at any time.
How to push your modification to your remote git repository
git push #use to push modification on an existing branch git push -u origin <my_New_branch> #Use to push a new branch. "-u" is the shortcut of --set-upstream
How to remove file from the staging area.
This command is the opposite of the git add command. Note that it will not reset your modification but only remove your files from the staging area.
git reset <specified path to a file> #if you want to only reset a file git reset #if you want to reset all the new or modified files.
How to delete all your modifications and go back to your local branch version.
Use this fonction only when you are sure that all the modifications you have done were not necessary.
git reset --hard origin <my_branch_name> #if you want to reset all the new or modified files.
How to manage local branches.
git branch #List all local branches git branch -d <my_branch_name> #delete a branch on your local machine. It will not delete the branch on the remote repository (gitlab, github etc). git branch <my_branch_name> #it will create a new branch but will not get on it like the checkout git command.
How to merge two branches.
This command will merge <my_branch_name> into your current branch.
git merge <my_branch_name>
Advanced git commands
There may be some cases where you will have to use the following git commands. But there are not commands that you will have to know to begin with. So be sure to only use this commands when you know what you are doing.
How to hold your modifications in memory.
git stash
I found this command useful when I wanted to switch to another branch but didn’t want my changes to disappear. Also, I didn’t want to push it to the local repository because those changes weren’t acceptable. This command will just hold my changes in the memory and if i wanted to get those changes, i would just have to type the following command on my branch or another one and BOOOM: It will appear again.
git stash pop
To get more information about the git stash command, you can click here.
How to list my commit history.
git log #List the commit of you current branch git log --all #List the commit of all your branches. git shortlog #List the commit of you current branch with their author to know who is responsible of what.
How to merge two branches (advanced)
git rebase <my_branch_name>
This command merge two branches in another way compared to the git merge command. It will rewrite the commit history while the git merge will just add the commits as the following one.
How to keep your git directory clean.
This is a builtin command to cleanup the untracked files. Be careful with this one, it deletes files permanently!
git clean -f
Conclusion
If you’ve read this far, you can be proud of you. Now i think you have an overview of what git is capable of and what you need to know to be proactive on your projects.
Thanks you and see you for the next articles!!